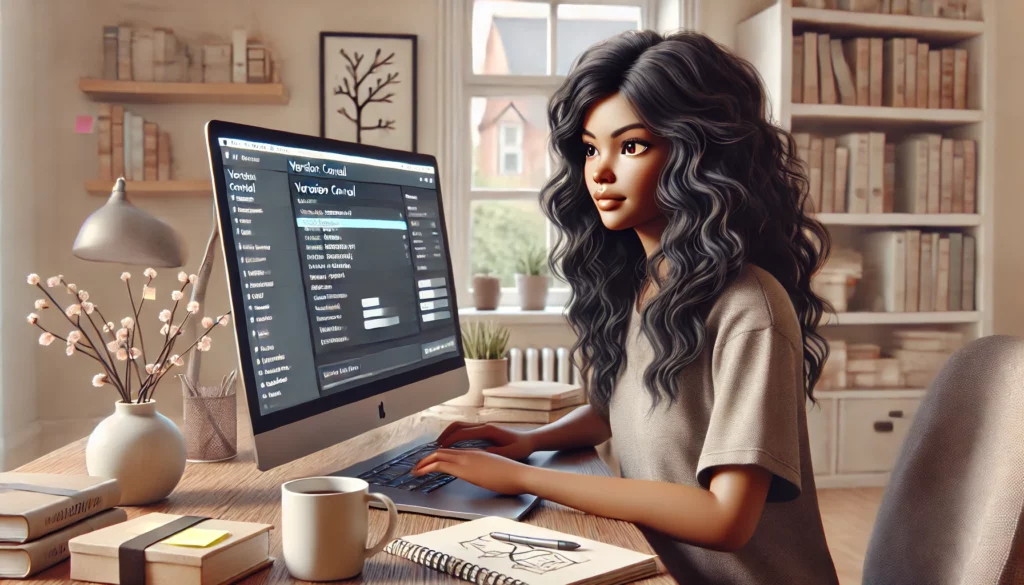
Why Git is Important for Version Control
Imagine you’re working on a software project with a team. Each member contributes code, and sometimes changes might conflict or break the build. Without a reliable system to manage these updates, chaos ensues. Git solves this by:
- Tracking Changes: Every modification is recorded with details about who made the change and when.
- Collaboration: Git enables multiple developers to work on the same project without overwriting each other’s work.
- Reverting Errors: If a mistake is made, Git allows you to roll back to a previous version.
- Branching and Merging: You can experiment with new features or fixes without disrupting the main codebase.
Getting Started with Git
1. Installation
Before using Git, you need to install it. Git is available for most operating systems:
- Windows: Download the installer from git-scm.com and follow the setup instructions.
- MacOS: Use Homebrew:
brew install git
. - Linux: Install via your package manager, e.g.,
sudo apt install git
on Debian/Ubuntu.
To verify the installation, run:
2. Configuring Git
Set your name and email, which will be associated with your commits:
You can view your settings with:
Core Concepts of Git
Repositories
A repository (repo) is where Git tracks changes. Repositories can be:
- Local: On your computer.
- Remote: Hosted on platforms like GitHub or GitLab.
Commits
A commit represents a snapshot of your project’s state at a specific point in time. Commits allow you to document and revert changes.
Branches
Branches enable parallel development. The default branch is typically main
or master
. You can create new branches for features, bug fixes, or experiments.
Working with Git: Step-by-Step
1. Initializing a Repository
To start tracking a project, navigate to the directory in your terminal and initialize Git:
This creates a .git
directory to store Git’s metadata.
2. Adding Files to Staging
After making changes to your project, you need to stage files for a commit. Use:
To stage all changes:
3. Committing Changes
Once files are staged, commit them:
Good commit messages are clear and concise, e.g., “Fixed login bug” or “Added user profile feature.”
4. Connecting to a Remote Repository
To collaborate, push your local changes to a remote repository. For example, on GitHub:
- Create a repository on GitHub.
- Link it to your local repo:
5. Pushing Changes
Send commits to the remote repository:
6. Pulling Changes
To get updates from the remote repo:
7. Branching and Merging
- Create a new branch:
- Switch to the branch:
- Combine changes from
feature-branch
intomain
:
8. Resolving Conflicts
When merging, conflicts can occur if two branches modify the same code. Git will highlight the conflicting areas in the files, and you must edit them manually.
After resolving, stage and commit the changes:
Advanced Git Commands
1. Viewing History
See all commits:
For a compact view:
2. Undoing Changes
- Undo unstaged changes:
- Undo staged changes:
3. Cloning Repositories
To copy an existing repo:
4. Stashing Changes
Temporarily save your changes without committing:
To retrieve them:
Collaborative Workflows
1. Forking and Pull Requests
- Fork a repository to your own GitHub account.
- Make changes in your copy, then propose them to the original repository using a pull request.
2. Code Reviews
Teams use pull requests to review and discuss changes before merging them into the main branch.
Best Practices
- Commit Often: Frequent commits make it easier to track changes and debug issues.
- Write Descriptive Messages: Commit messages should explain the purpose of the change.
- Use Branches: Isolate features or fixes in separate branches to avoid disrupting the main codebase.
- Pull Before Pushing: Always pull the latest changes from the remote repository before pushing your commits to avoid conflicts.
Common Mistakes to Avoid
- Committing Sensitive Data: Never commit passwords or API keys.
- Ignoring Merge Conflicts: Take the time to resolve conflicts carefully.
- Not Using .gitignore: Use a
.gitignore
file to exclude unnecessary files like temporary builds or logs.
Conclusion
Mastering Git is essential for effective version control in software development. With Git, you can track changes, collaborate seamlessly, and experiment with confidence. Whether you’re a solo developer or part of a large team, understanding Git workflows and best practices will greatly enhance your productivity.
Git may seem daunting at first, but with consistent practice, it becomes second nature. Start with the basics, experiment with branches and merges, and soon you’ll be managing your codebase like a pro.
So, open your terminal, create a repository, and embrace the power of Git today!